티스토리 뷰
Sample Error Code :
https://wandbox.org/permlink/oAXbYnkKE5BR7Wig
[Wandbox]三へ( へ՞ਊ ՞)へ ハッハッ
wandbox.org
#include <iostream>
#include <memory>
#include <thread>
std::shared_ptr<int> g;
void read_g()
{
std::shared_ptr<int> x;
long sum = 0;
for (int i = 0; i < 1000 * 1000; ++i)
{
x = g;
sum += *x;
}
printf("sum = %ld\n", sum);
}
void write_g()
{
for (int i = 0; i < 1000 * 1000; ++i)
{
auto n = std::make_shared<int>(42);
g = n;
}
}
int main()
{
g = std::make_shared<int>(42);
std::thread t1(read_g);
std::thread t2(write_g);
t1.join();
t2.join();
}
Reference :
https://www.slideshare.net/zzapuno/multithread-sharedptr
Multithread & shared_ptr
How to avoid data race using shared_ptr
www.slideshare.net
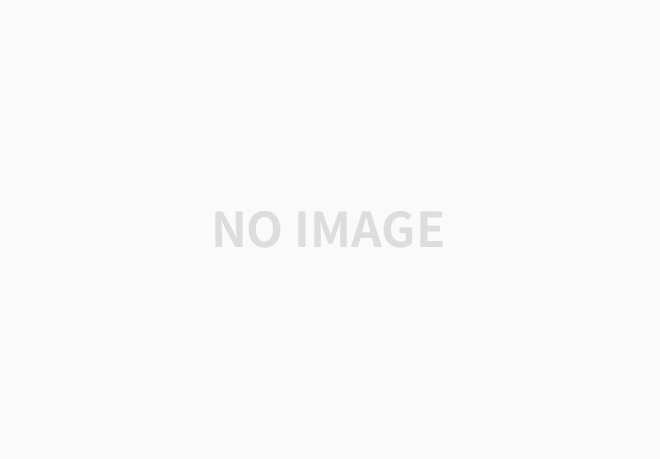
Shows an error when using shared_ptr in a multithreaded environment.
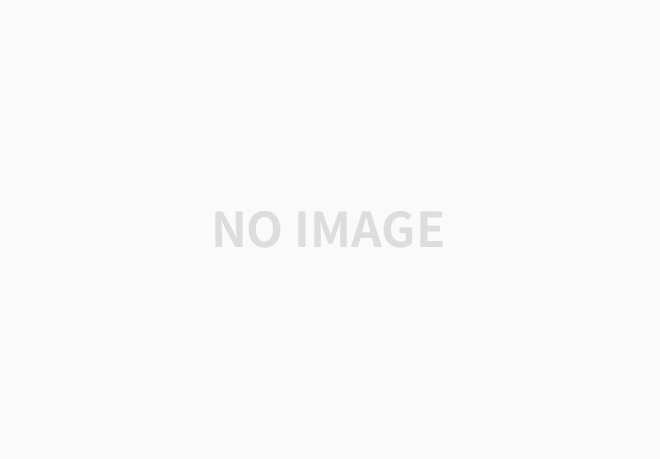
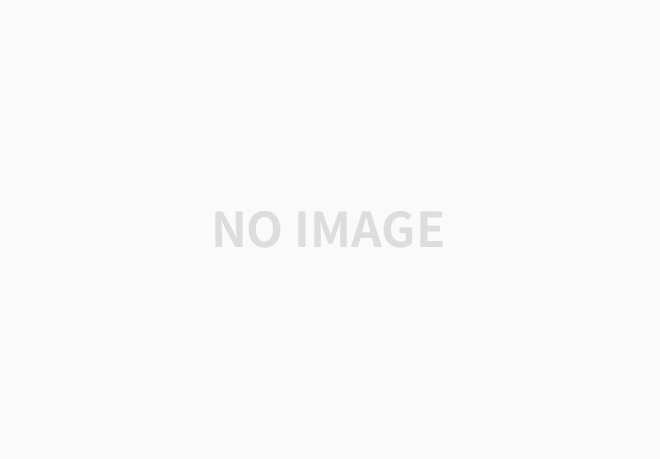
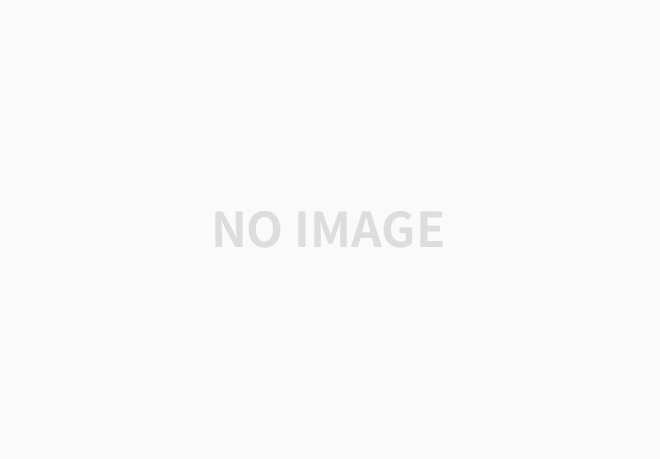
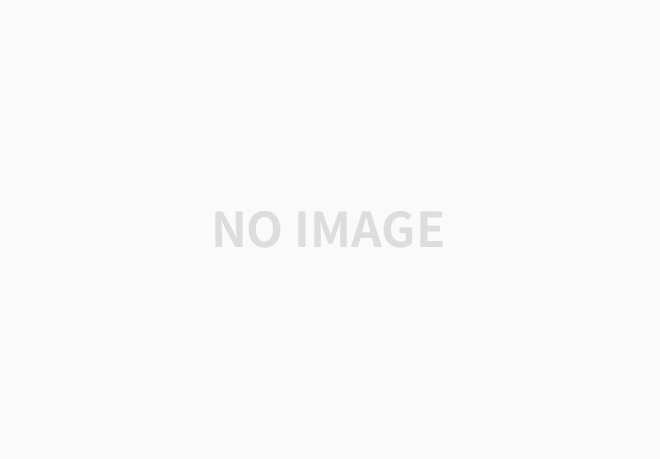
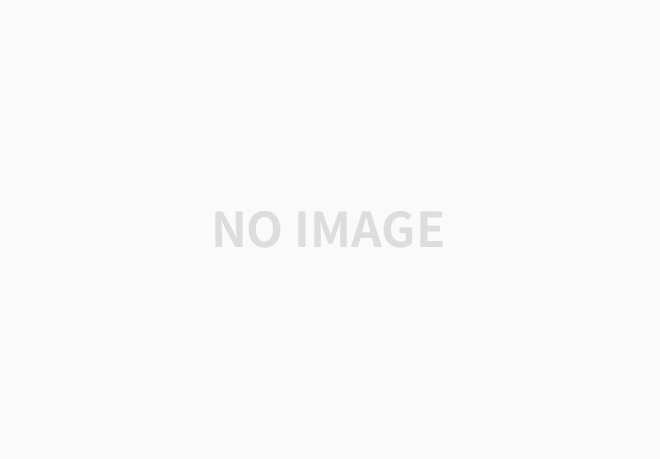
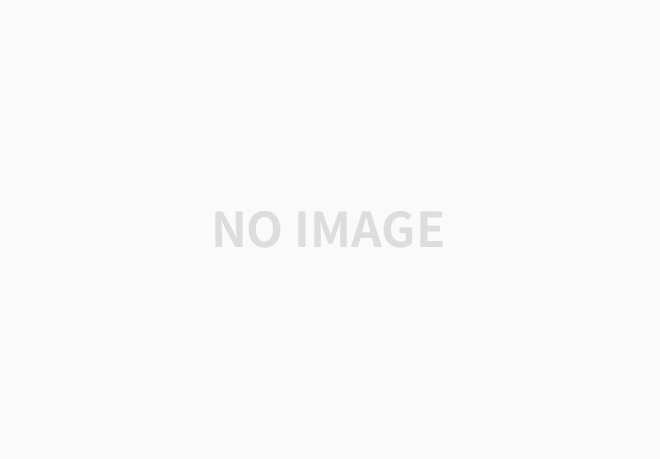
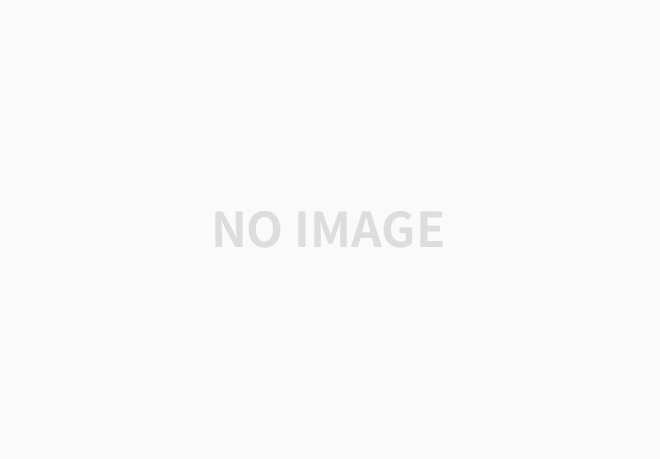
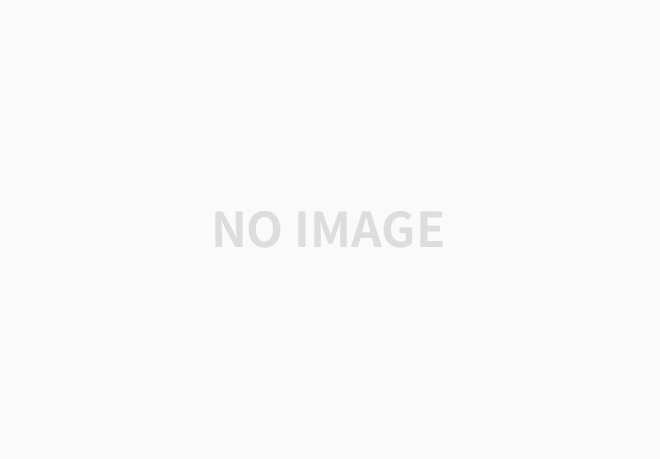
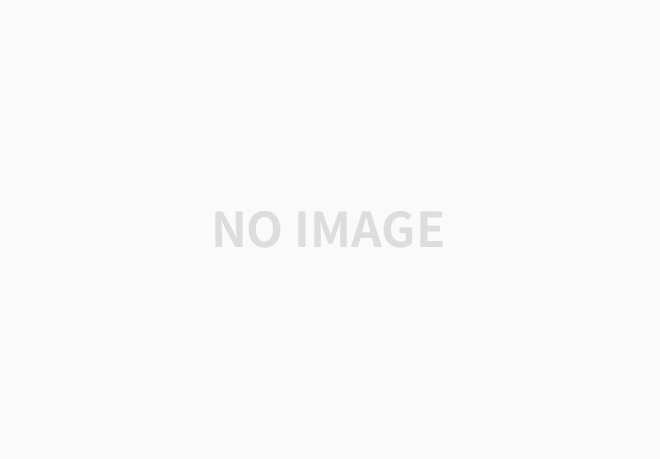
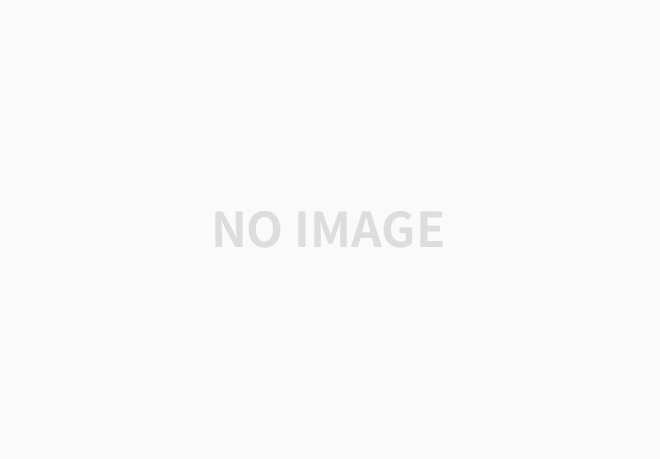
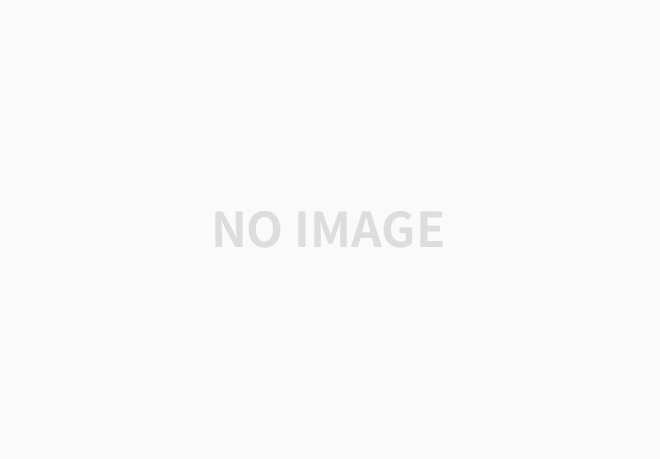
To solve this problem, atomically load and store shared_ptr.
Sample Correct Code :
wandbox.org/permlink/OWNnFaPmXkbmdBAl
[Wandbox]三へ( へ՞ਊ ՞)へ ハッハッ
wandbox.org
#include <iostream>
#include <memory>
#include <thread>
std::shared_ptr<int> g;
void read_g()
{
std::shared_ptr<int> x;
long sum = 0;
for (int i = 0; i < 1000 * 1000; ++i)
{
x = std::atomic_load(&g);
sum += *x;
}
printf("sum = %ld\n", sum);
}
void write_g()
{
for (int i = 0; i < 1000 * 1000; ++i)
{
auto n = std::make_shared<int>(42);
std::atomic_store(&g, n);
}
}
int main()
{
g = std::make_shared<int>(42);
std::thread t1(read_g);
std::thread t2(write_g);
t1.join();
t2.join();
}
If there is a mistake, please contact me
'개발 > 일상' 카테고리의 다른 글
키보드 입력으로 특정 위치 마우스 클릭 프로그램 (윈도우) (0) | 2020.10.17 |
---|---|
오픈소스 cockroach db 기여 후기 (0) | 2020.07.28 |
Scheduler와 Dispatcher의 차이 (0) | 2020.04.15 |
visual studio 2019 c++ mysql connector8.0 (3) | 2020.01.20 |
2020 넷마블 신입공채 '넷마블 네오 게임 서버' 최종 합격 후기 (7) | 2019.12.17 |
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
TAG
- 에러 위치 찾기
- chrome-extension
- vrpit
- hole-punching
- Reciprocal n-body Collision Avoidance
- 면접
- red underline
- 영상 픽셀화 하기
- 카카오
- boost
- mysql
- print shared_ptr class member variable
- C++
- SuffixArray
- 봄날에 스케치
- Visual Studio
- ad skip
- vr핏
- RVO
- Golang
- set value
- 코어 남기기
- Quest2
- Obstacle Avoidance
- 클래스 맴버 변수 출력하기
- 잘못된 빨간줄
- 우리는 vr핏이라고 부릅니다
- shared_from_this
- cockroach db
- it's called a vrpit
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
글 보관함